For one reason or another I love the "minimalist wallpapers" you can find for your desktop (not that my desktop is ever clean enough to warrant what that phrase implies). There are a lot of sites out there that publish a specific one I am overly fond of which looks like a stylized mountain range drifting into the fog. I like this one so much, I used it for my AppStore screenshots for ZeroScan.
After using this image a lot, and it being a little too long since I wrote any "creative" code, I decided I wanted to replicate this image, and see if I could iterate on the concept. So I decided to write some code and see where I landed.
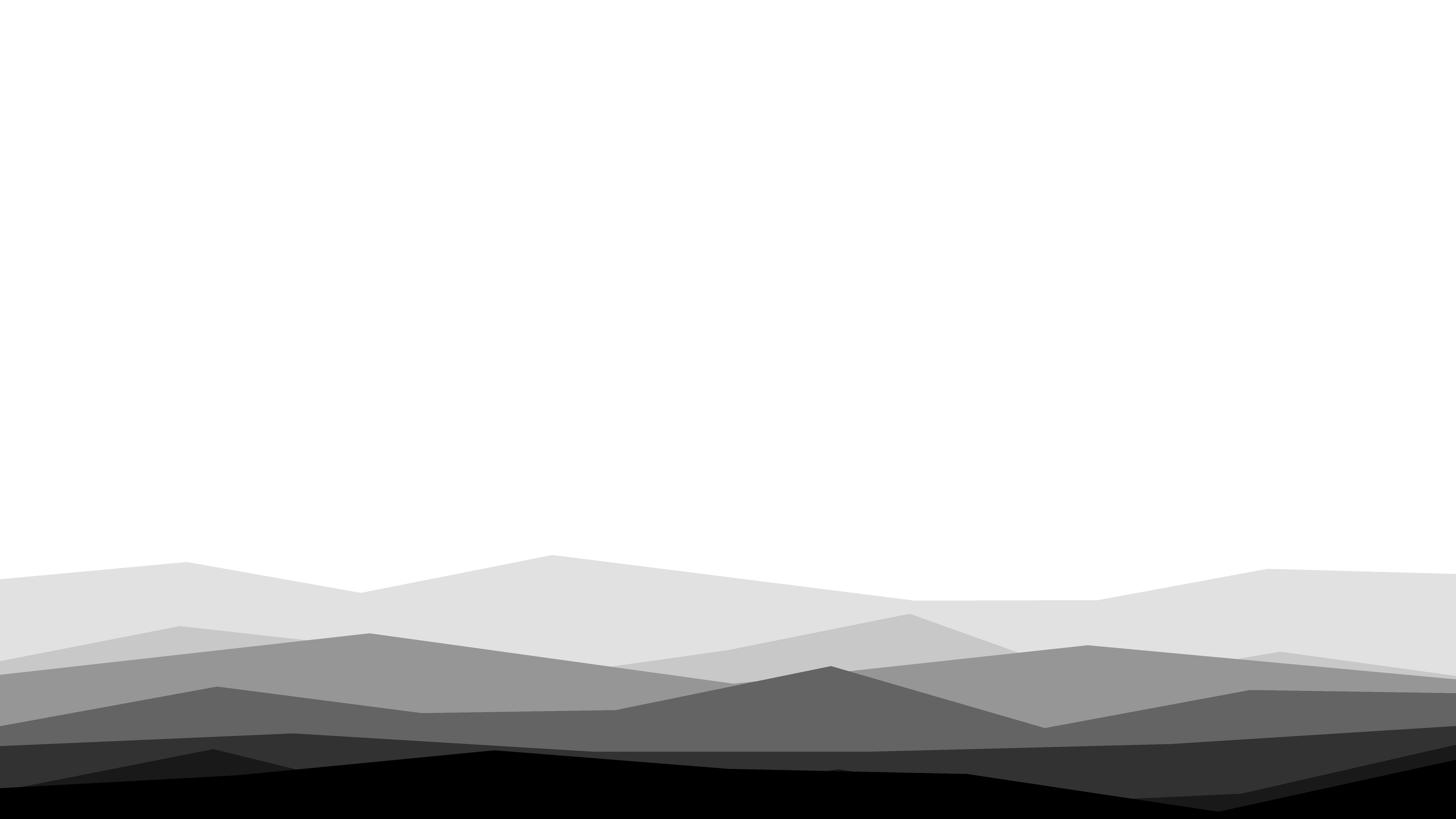
After a little bit of fiddling with python I had replicated the base image to my satisfaction. I used Python, numpy, and Pillow for this, which I know might seem like an odd choice, especially when Processing and OpenFrameworks are so readily available, but I am using python day-to-day and it was what was closest to the surface.
In less than 50 lines I had achieved my goal of 4K resolution infinitely generated minimalist wallpapers. The code wasn't wonderful, but it was effective. My approach was to calculate a line of points across the screen, close it in as a polygon, fill that shape with one level of grey, then repeat the drawing process slightly lower, and in a slightly darker color.
Here is the function that actually generates the line moving across the screen:
This level of controlled chaos left me with some nice options, but I wanted to push things a little further. So I decided to add color.
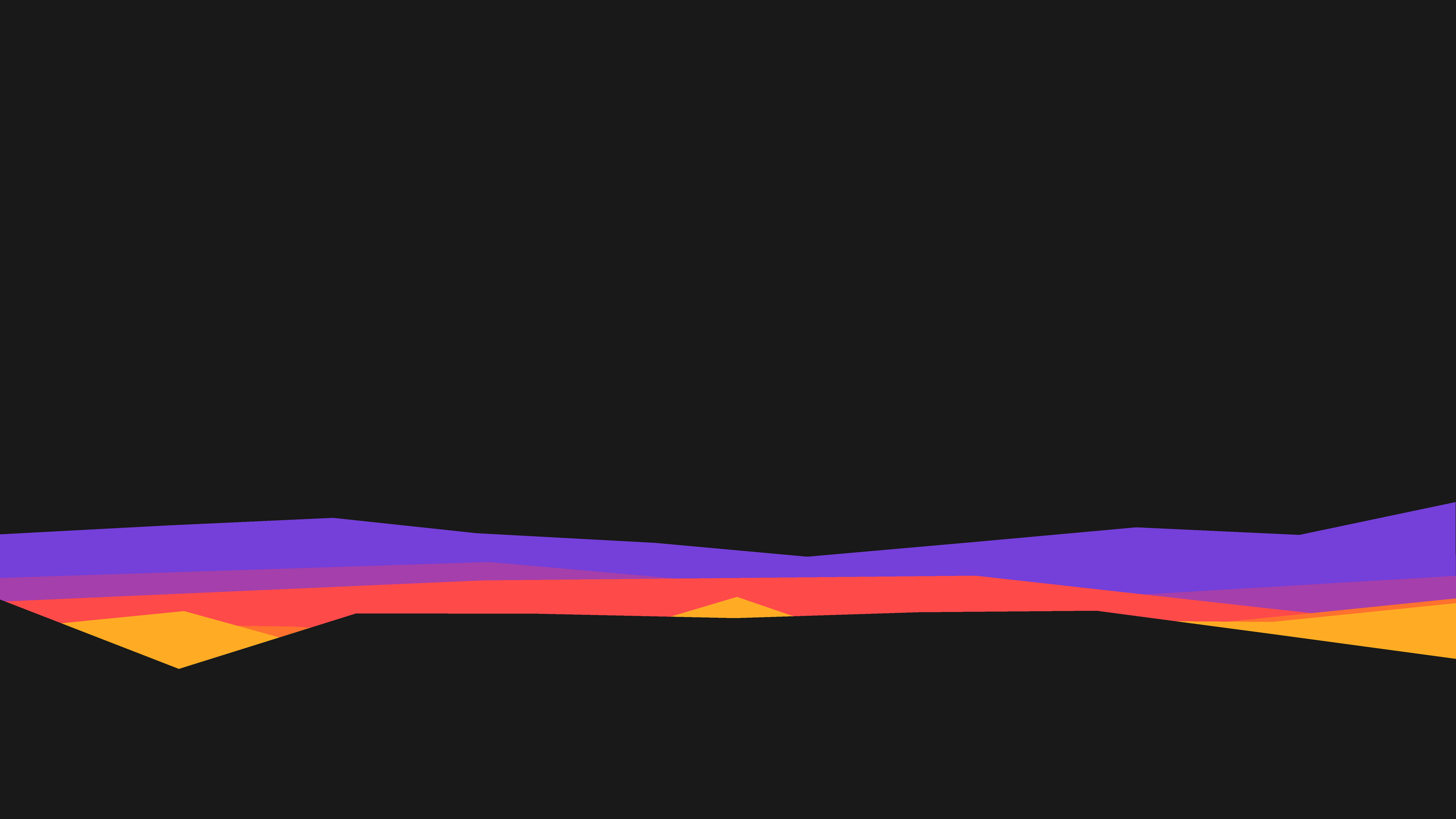
Adding color lead me to the realization that if I moved the lines up on the screen, and repeated the background color as the last polygon I was left with a crack / reveal look that I am very happy with. The calm dark grey fragments slightly to reveal a minimal layering of color.
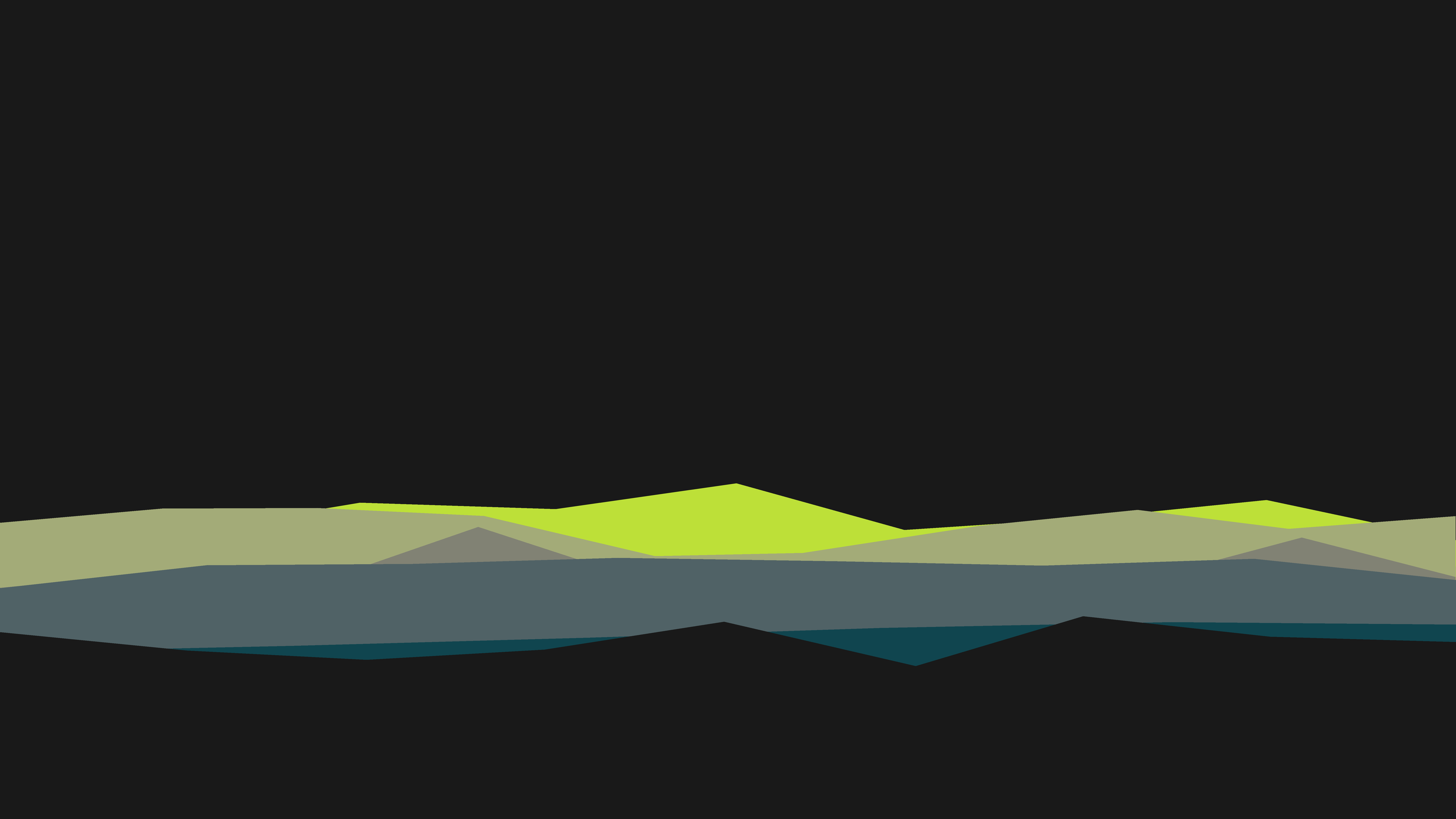
While I may have wandered slightly from my original goal, I am happy with the results. I want to make more little explorations like this, and attempt to document them. Towards that end I've setup a repo with this code, where in future I will hopefully be adding more graphics experiments: gitlab.com/JordanParsons/gfx.